제일 작은 수 제거하기
문제정의
배열에서 제일 작은 수를 제거하여 출력한다.
문제풀이
전체 코드는 다음과 같다. 1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29import java.util.*;
public class RemoveMin {
//프로그래머스 문제풀이 level1 제일 작은 수 제거하기
public static void main(String[] args)
{
int[] arr = {4,3,2,1};
ArrayList<Integer> sorted_list = new ArrayList<Integer>();
for(int n : arr)
sorted_list.add(n);
ArrayList<Integer> unsorted_list = new ArrayList<Integer>();
unsorted_list = (ArrayList<Integer>)sorted_list.clone();
Collections.sort(sorted_list);
Integer min = sorted_list.get(0);
unsorted_list.remove(min);
int[] answer = new int[unsorted_list.size() == 0 ? 1 : unsorted_list.size()];
if(answer.length == 1)
answer[0] = -1;
else
{
for(int i = 0; i < unsorted_list.size(); i++)
answer[i] = Integer.parseInt(unsorted_list.get(i).toString());
}
}
}1
2
3
4
5
6
7
8int[] arr = {4,3,2,1};
ArrayList<Integer> sorted_list = new ArrayList<Integer>();
for(int n : arr)
sorted_list.add(n);
ArrayList<Integer> unsorted_list = new ArrayList<Integer>();
unsorted_list = (ArrayList<Integer>)sorted_list.clone();
Collections.sort(sorted_list);1
2Integer min = sorted_list.get(0);
unsorted_list.remove(min);1
2
3
4
5
6
7
8int[] answer = new int[unsorted_list.size() == 0 ? 1 : unsorted_list.size()];
if(answer.length == 1)
answer[0] = -1;
else
{
for(int i = 0; i < unsorted_list.size(); i++)
answer[i] = Integer.parseInt(unsorted_list.get(i).toString());
}
테스트
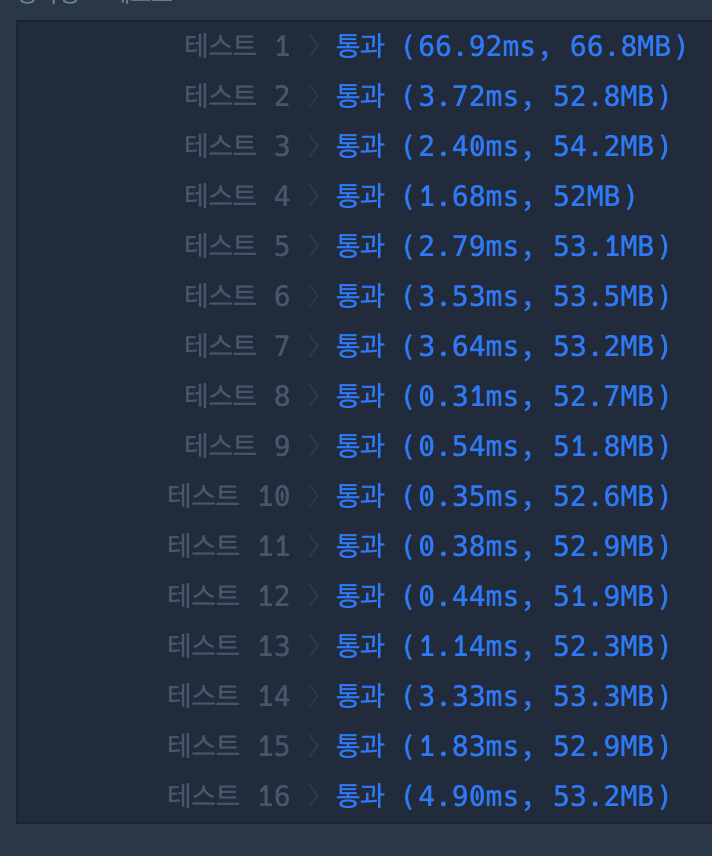
테스트케이스는 전부 통과하였지만 만약 테스트케이스가 [1,1,3]인 경우 내 알고리즘은 틀리게 된다. 그래도 통과가 된걸보니 그러한 케이스는 없었던 것 같다.